Arrays in Go lang
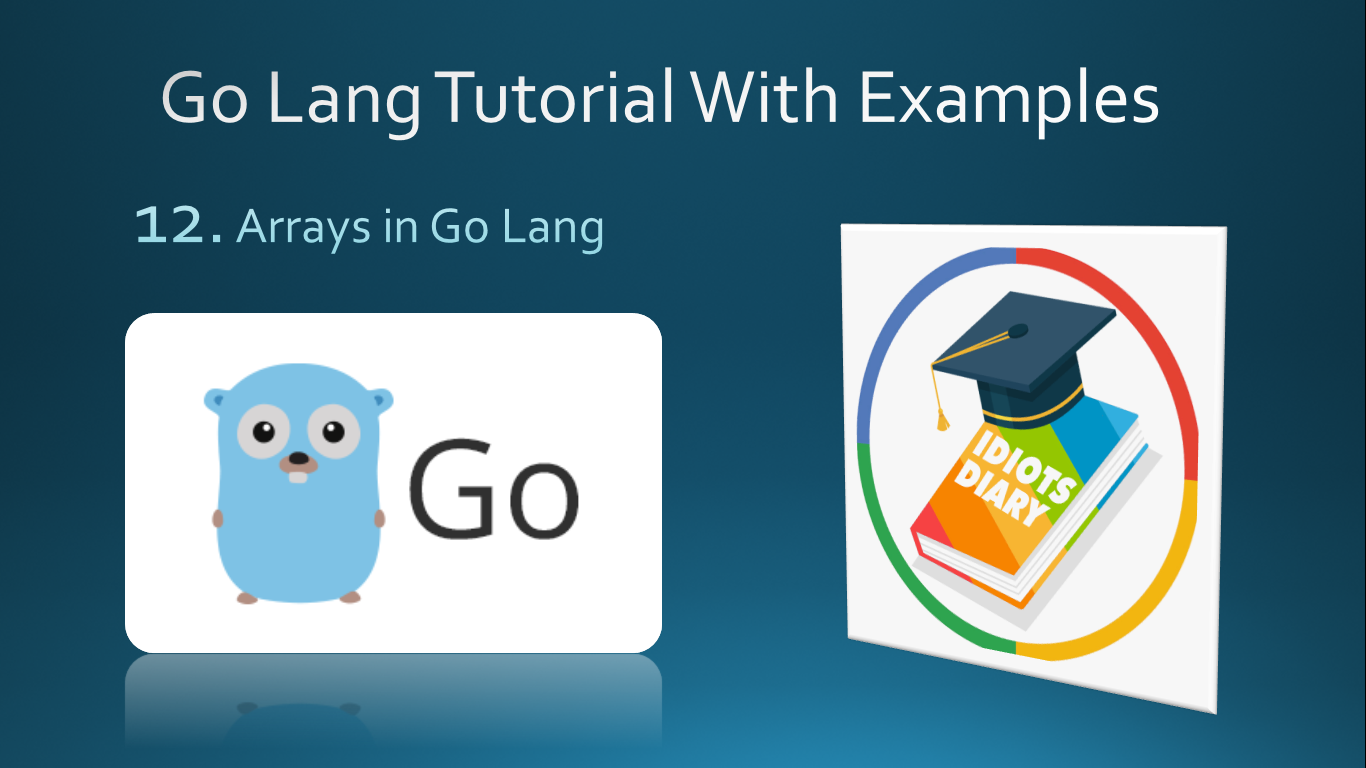
What is Array An array is the collection of similar type of elements. An array is the collection of fixed length. Array elements are stored in contiguous memory locations. All the array elements share the common name, but each element is uniquely identified their index or subscript, so the array is also called a subscript variable. Array index always starts with 0. Creating an array var arr [5]int Note: In the above program snippet, arr is an array of 5 integers. By default, all the array elements are initialized with a default value. Default Values Data Type Default Value Int(s) 0 Float(s) 0.0 bool(s) false Objects nil Note: Following example demonstrate the default values for array elements. Example-1 package main import "fmt" func main() { var arr1 [5]int var arr2 [5]float32 var arr3 [5]string var arr4 [5]bool fmt.Pr...