Program to check a year is leap year or not.
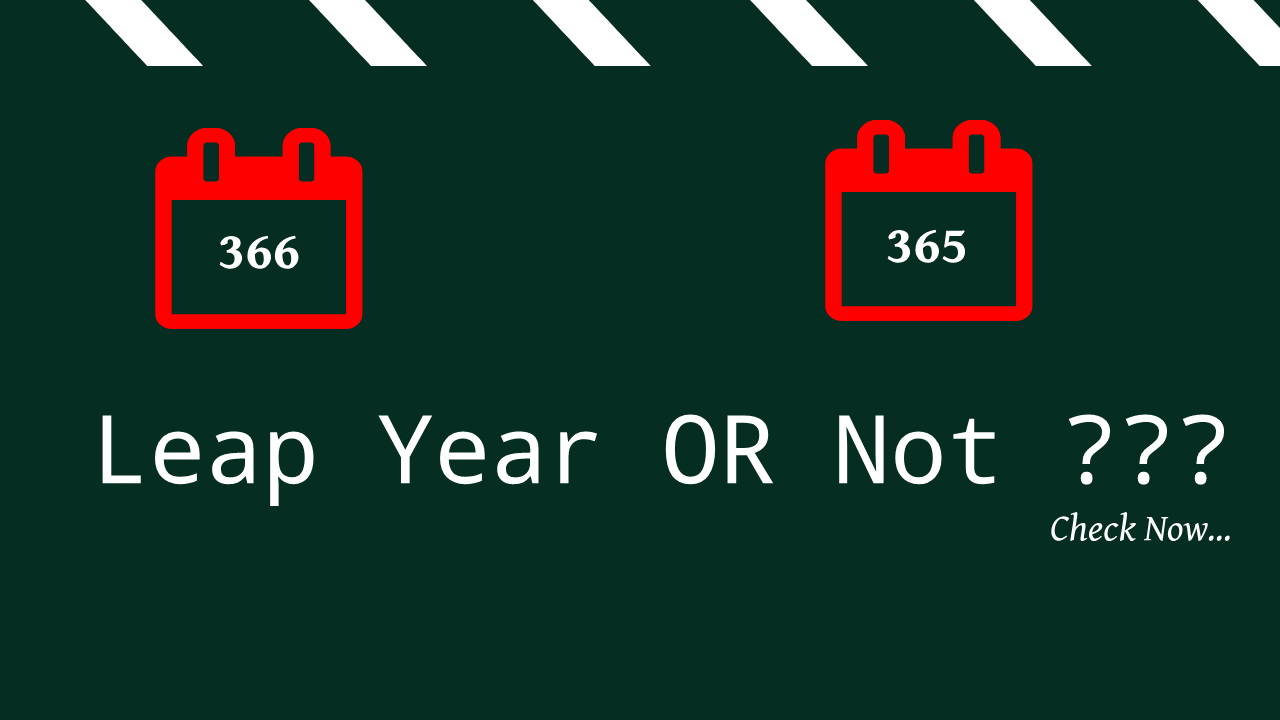
Problem Description
- If a year is divisible by 4, then it is called leap year.
- But all the centuries are divisible by 4, so to check a century is a leap year or not, we check divisibility by 400 of a year.
- If a century is divisible by 400 then the century is called leap year otherwise not.
Basic Logic Snippets (In C)
if(year % 100 == 0) {
if (year % 400 == 0) {
printf("%d is a leap year",year);
} else {
printf("%d is not a leap year",year);
}
}else {
if(year % 4 == 0) {
printf("%d is a leap year",year);
} else {
printf("%d is not a leap year",year);
}
}
Output
Test-1
Enter any year: 1996
1996 is a leap yearTest-2
Enter any year: 1600
1600 is a leap yearTest-3
Enter any year: 1800
1800 is not a leap year
Improved your logic
Note: Generate wrong output in some cases, but helpful to understand upcoming logic
if(year % 100 == 0 && year % 400 ==0 || year % 4 == 0) {
printf("%d is a leap year",year);
} else {
printf("%d is not a leap year",year);
}
- Above program generates correct output in the case of 1600 and 1996 but generates wrong output in the case of 1800
- In the case of 1800, year is century but not divisible by 400, so condition (year%100 == 0 && year%400 ==0), evalutes as false. and the next condition combined with OR(||) operator means (year%4==0) is evaluted and evalutes true.
- But the second condition (year%4==0) should be evalutes only when year is not a century so the above program snippet generates wrong output in some cases.
- Above program snipped problem can be fixed as shown in the following program snippet.
if(year % 100 == 0 && year % 400 ==0 || year%100!=0 && year % 4 == 0) {
printf("%d is a leap year",year);
} else {
printf("%d is not a leap year",year);
}
- As shown in the above program snippet now condition (year%4==0), only evalutes if year is not a century and it confirmed by the condition year%100!=0
- But as we know, 100 is one of the factor of 400, so if a thing is divisible by 400 then also divisible by 400, so the final version of above program snippet is as given below.
if(year % 400 ==0 || year%100!=0 && year % 4 == 0) {
printf("%d is a leap year",year);
} else {
printf("%d is not a leap year",year);
}
C Program
#include<stdio.h>
int main() {
int year;
printf("Enter any year: ");
scanf("%d",&year);
if(year % 400 ==0 || year%100!=0 && year % 4 == 0) {
printf("%d is a leap year",year);
} else {
printf("%d is not a leap year",year);
}
return 0;
}
C++ Program
#include<iostream>
using namespace std;
int main() {
int year;
cout << "Enter any year: ";
cin >> year;
if(year % 400 ==0 || year%100!=0 && year % 4 == 0) {
cout << year << " is a leap year";
} else {
cout << year << " is not a leap year";
}
return 0;
}
Java Program
import java.util.Scanner;
class IsLeapYear {
public static void main(String args[]) {
int year;
Scanner sc = new Scanner(System.in);
System.out.print("Enter any year: ");
year = sc.nextInt();
if(year%400 ==0 || year%100!=0 && year%4==0) {
System.out.println(year+" is a leap year");
}else {
System.out.println(year+" is not a leap year");
}
}
}
Go Lang
package main
import "fmt"
func main() {
var year int
fmt.Print("Enter any year: ")
fmt.Scan(&year)
if year % 400 == 0 || year % 100 != 0 && year % 4 == 0 {
fmt.Print(year," is a leap year")
} else {
fmt.Print(year," is not a leap year")
}
}
PHP
if(isset($_POST['sub'])) {
$year = $_POST['year'];
if($year % 400 == 0 || $year % 100 != 0 && $year % 4 == 0) {
echo $year." is a leap year";
} else {
echo $year." is not a leap year";
}
}
Comments