Program to Compute real roots of a quadratic expression
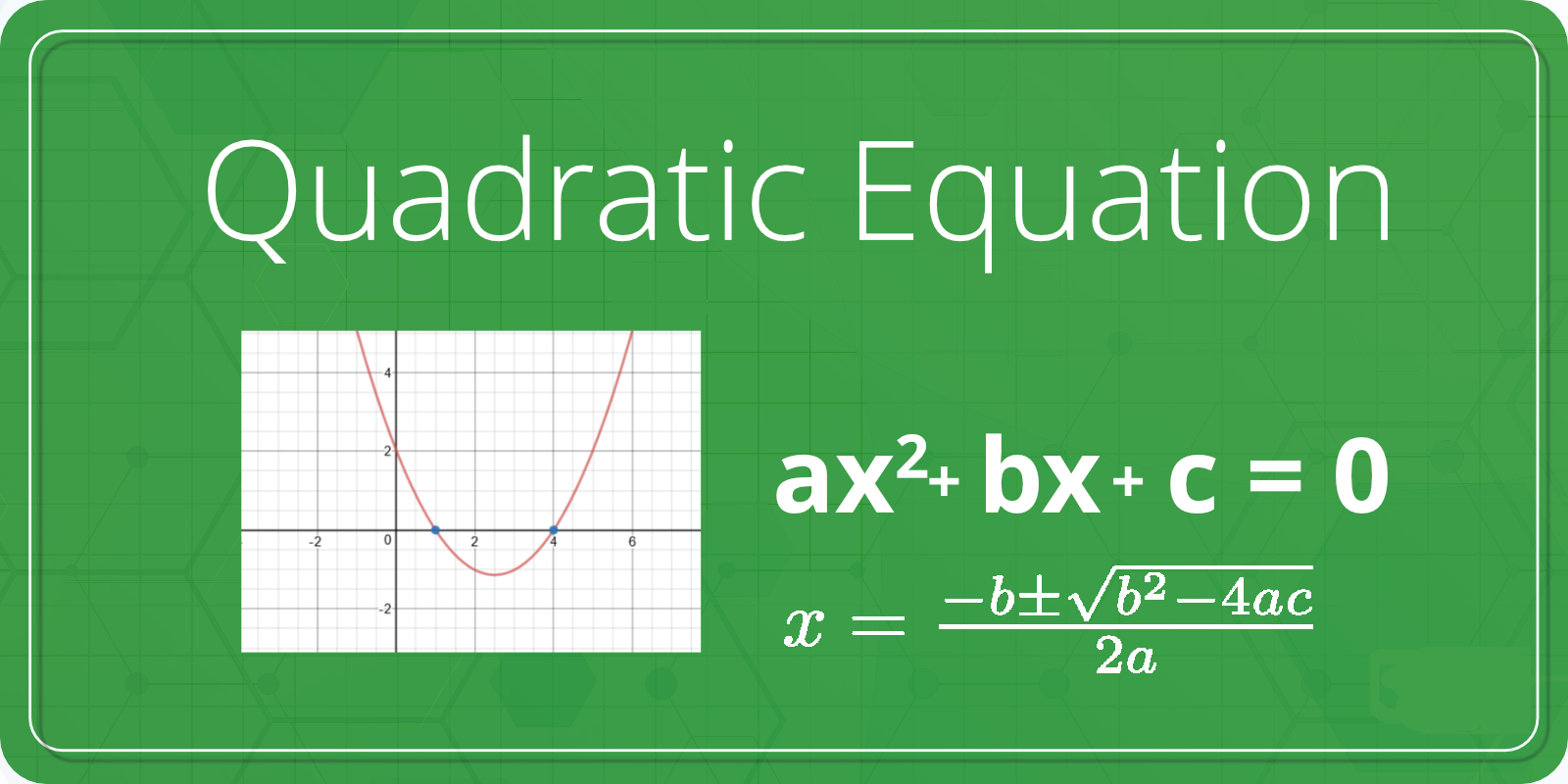
Problem Description Program to find the real roots of a quadratic equation as given below ax 2 + bx + c is a quadratic equation, we need to find the real root for these type of expressions, the formula to find the root is as given below Where Discriminant of a Quadratic represented by D = b 2 – 4ac, which reveals the type of roots as per the following conditions If D >0, the roots would be real If D = 0, then both roots would be equal If D < 0, the roots would be imaginary. C Program #include<stdio.h> #include<math.h> int main() { int a,b,c; float d,r1,r2; printf("Enter 3 constant a,b,c: "); scanf("%d%d%d",&a,&b,&c); d = b * b - 4 * a * c; if (d < 0) { printf("Roots are imagenary"); }else { r1 = (b + sqrt(d)) / (2 * a); r2 = (b - sqrt(d)) / (2 * a); printf("Root1 = %.2f\nRoot2 = %.2f",r1,r2); } return 0; } C++ program #include<iostream> #include<math.