Literals in Java
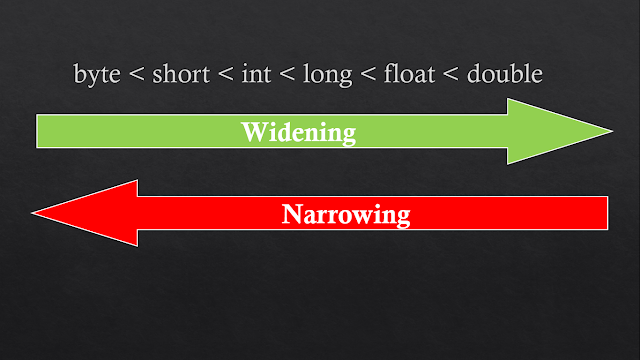
Literals in Java int a = 5; In the above expression, a is a variable but 5 is literal. Literal can be of many types, we will discuss all types of literal one by one. Integral Literal Any integer constant is treated as integral literal. it can be written in 3 formats. int a = 10; (decimal format) int a = 010; (octal format, equivalent to 8) int a = 0x10; (hexadecimal format, equivalent to 16) Note: Octal constants can contain digits from 0 to 9. Hexadecimal constants can contains digits from 0 to 9 and a to f, where a to f can be uppercase or lowercase. Example - 1: int a = 10; ( ✓ ) int a = 0678; (✕) // Compile time error, number too large int a = 0777; ( ✓ ) int a = 0xace; ( ✓ ) int a = 0xBeer; (✕) // Compile time error, number too large Example - 2: int a; a = 10 + 010 + 0x10; System.out.println(a); // 34, because 010 = 8 and 0x10 = 16 so 10 + 8 + 16 Important Notes We(programmer) have option to write integer constant in dec