Looping in go lang
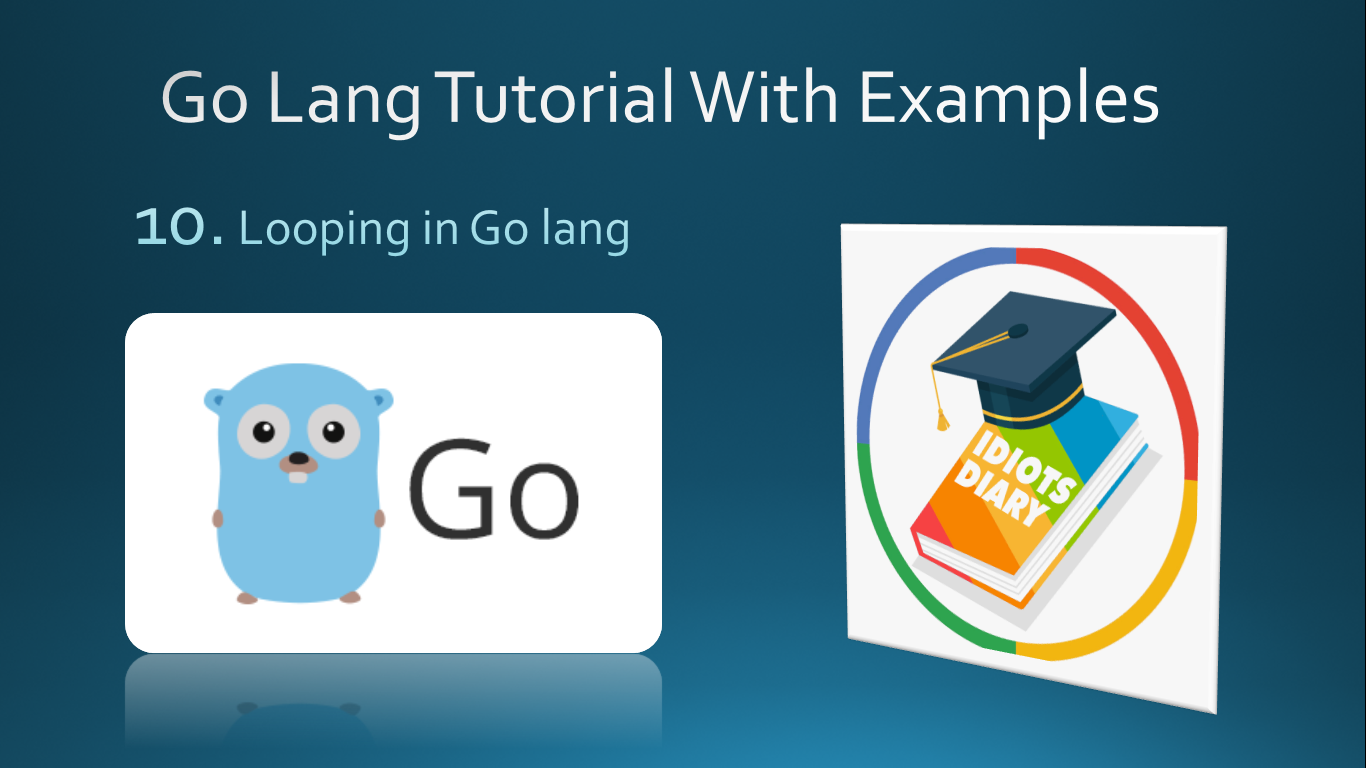
Loop is a structure with the block of statements can be repeated for the finite number of times. Till Now we have learned the programs that execute sequentially, Now we are going to learn control structures which change the normal flow of the program and helps you to create more meaningful and compact program structure. To understand more, suppose we want to print from 1 to 10 number counting, each number should be printed in the new line. Program to print numbers from 1 to 10 package main import "fmt" func main( ) { fmt.Println(1) fmt.Println(2) fmt.Println(3) fmt.Println(4) fmt.Println(5) fmt.Println(6) fmt.Println(7) fmt.Println(8) fmt.Println(9) fmt.Println(10) } Another compact way to create this program use loop. The following example creates this program using the loop. Program package main import "fmt" func main() { i := 1 //initialization for i ...